What is Selenium WebDriver: Exploring Advanced Use Cases
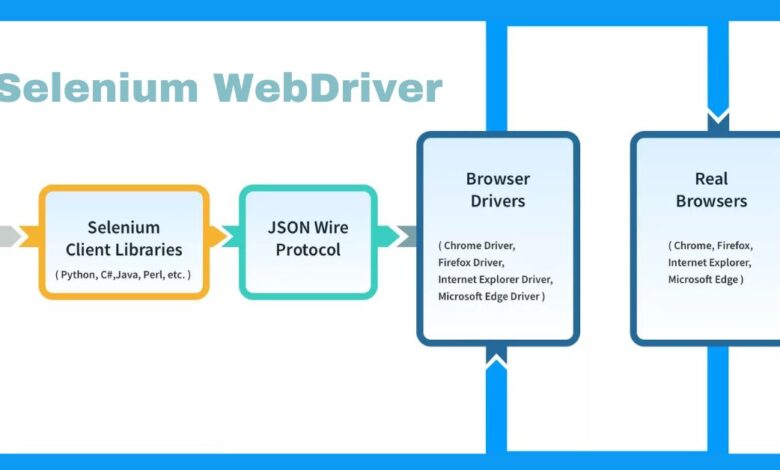
WebDriver is a user-centric interface that allows you to control a browser locally or on a multiple device. It is a universal protocol that functions across diverse programming languages and platforms, enabling you to remotely guide a browser’s activities, such as finding and modifying DOM components or managing customer agent behavior.
Now part of the Selenium project, Selenium WebDriver combines browser control code and language bindings, often called WebDriver. This tool is essential for automating browser testing across distinct Oss and browsers, guaranteeing a seamless user experience. Its important to know about what is Selenium WebDriver? before you get started with the tool.
As the online world progresses, delivering high-end user experiences becomes vital. For sites and web applications, it is crucial to confirm a smooth end-user experience. Using automation testing tools is the go-to technique for testing your product on multiple OSs and browsers. Selenium, supporting diverse languages such as JavaScript, C#, Java, Ruby, and more, is a pristine tool for big companies aiming to automate their software test procedures.
In this article, we will give core insight into advanced use cases of Selenium WebDriver, demonstrating how this versatile tool can be leveraged to improve the effectiveness and coverage of your test automation efforts.
What is Selenium WebDriver?
Selenium WebDriver is a dynamic automated tests tool that enables you to programmatically control web browsers. It offers a user-friendly interface to communicate with web components and execute operations like entering text, clicking buttons, and navigating between web pages.
It supports multiple programming languages and is vital for automating recurring jobs, validating web apps, and confirming they function smoothly and flawlessly across different platforms and browsers.
Crucial Features of Selenium WebDriver
Selenium is an extensively used open-source test framework that comes with numerous features, addressing the needs of modern automation testing. What is Selenium? It is a versatile tool that offers several key capabilities, making it a popular choice for web automation:
- Supported Languages for Convenience: It supports several languages, such as C#, Python, Java, JavaScript, and Ruby. This flexibility enables QA automation testers to utilize their ideal language without learning extra ones.
- WebDriver API for Browser Control: The WebDriver Application Programming Interface allows you to control web browsers through code, enabling activities such as filling out forms and clicking links. This makes generating scripts that can run from the command line or be incorporated with other tools effortlessly.
- Testing Across Diverse Web Browsers: Selenium Web Driver allows tests on several browsers such as Mozilla Firefox, Google Chrome, and Internet Explorer. This guarantees that web apps function perfectly across various devices and browsers.
- Generating Dynamic Automated Tests: By using Se (Selenium) APIs in diverse languages, integration competencies, cross-browser support, and personalizing options, QA Engineers can create automated tests based on their project’s necessities. This approach saves extra effort and time by conducting parallel testing across several devices using Grid Distribution.
- Effective Parallel Tests & Grid Distribution: Parallel testing allows you to conduct several tests simultaneously on diverse machines, and Grid Distribution aids in distributing tests across multiple devices. This expedites test implementation when dealing with a huge number of tests.
- Customization with User Plugins and Extensions: Users can improve Selenium WebDriver’s competencies by installing user extensions or plugins, adding new traits, or modifying existing ones based on particular project requirements.
- Incorporation with Other Frameworks: Selenium Web Driver incorporates other testing frameworks, such as TestNG or JUnit, to generate automated tests for your web app.
- Test Reports & Dashboards: Selenium creates comprehensive test reports & real-time dashboards, assisting QA testers access progress and rapidly detecting flaws in automated tests.
When to make use of Selenium WebDriver?
Let’s discover particular examples of Selenium WebDriver being extremely effective.
- Cross-Browser Tests: Check that web apps maintain constant appearance as well as functionality across browsers such as MS Edge, Mozilla Firefox, Google Chrome, and Safari. Discover cross-browser compatibility advantages and traits in a tutorial hub.
- Cross-Platform Tests: Evaluate web app performance on Windows, Linux, and macOS OSs, confirming a smooth and flawless user experience across platforms.
- Functional Tests: Automates user deeds like filling out forms and clicking buttons to ensure that web apps function appropriately. This includes checking the projected outcomes to authenticate the app’s behavior.
- UI/UX Tests: Automates assessment to confirm your app’s visual components and layout remain steady, providing a positive user experience.
- Data-Driven Tests: Optimizes test effectiveness by using automatically created or database-pulled test data. Conduct similar testing with various datasets to cover a set of circumstances and scenarios.
- Regression Tests: Guarantees the continued functionality of current traits even when new ones are presented. This protects against unintended adverse effects during software/ app updates.
- Parallel Tests: Saves time by incorporating tools such as Selenium Grid to conduct testing concurrently on several browsers. This expedites the test procedure and improves efficiency.
- End-to-End (E2E) Tests: Mimics fundamental user relations across multiple app sections to validate a seamless and cohesive user experience from start to end.
- Complicated User Flows Simulates and tests complex user interactions that necessitate repetition, confirming that intricate user pathways are authenticated systematically.
- Intricate Scenarios Management: Mitigates challenges such as iframes, pop-ups, alerts, and dynamic content using WebDriver’s flexible capabilities. It confirms full testing under varied scenarios.
- CI Pipelines Incorporation: Incorporate into your CI/CD pipeline to automate tests with every single code change. This incorporation gives consistent code quality during development.
Key Techniques for WebDriver Automation
Below are three techniques for automation:
1. Managing Dynamic Web Components
Web apps often include robust content that fluctuates without refreshing the web page. Managing such components necessitates advanced methods:
- Explicit Waits: Utilize WebDriver’s WebDriverWait class to wait for some situations to be met before proceeding with activities.
- JavaScript Executor: Utilize JavaScript to interact with components that aren’t easily available through standard WebDriver commands.
2. Automating File Downloads & Uploads
Automating file downloads & uploads can be tricky but is attainable with WebDriver:
- File Downloads: Configure settings of the particular web browser to automatically manage file downloads.
- File Uploads: Utilize the sendKeys technique to upload files by setting the file path straight to the file input component.
WebElement uploadElement = driver.findElement(By.id(“upload”));
uploadElement.sendKeys(“/path/to/file”);
3. Working with Windows and Frames
Handling frames and multiple windows requires switching the WebDriver context:
- Handling Multiple Windows: Utilize driver.getWindowHandles() to shift between diverse web browser windows.
- Swapping to Frames: Utilize driver.switchTo().frame() to communicate with components within an iframe.
String mainWindow = driver.getWindowHandle();
Set<String> allWindows = driver.getWindowHandles();
for (String window: allWindows) {
if (!window.equals(mainWindow)) {
driver.switchTo().window(window);
// Perform actions on the new window
driver.close();
}
}
driver.switchTo().window(mainWindow);
What are the Best Practices to Make Use of Selenium WebDriver?
Let’s check out some of the effective guidelines to confirm the effective use of Selenium WebDriver:
1. Leverage Explicit and Implicit Waits
Synchronization problems are common in test automation. Selenium WebDriver provides implicit and explicit waits to manage these issues and confirm that components are accessible before running actions on them.
- Explicit Wait:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“elementId”)));
- Implicit Wait:
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
2. Follow the Page Object Model Design Pattern
Implementing the POM pattern can improve test maintenance & regulate code repetition by extracting the test logic from the page-centric code. Every page (within the app) is denoted by a specific page class, which comprises the components precise to that web page.
For Instance:
public class LoginPage {
private WebDriver driver;
@FindBy(id = “username”)
private WebElement usernameField;
@FindBy(id = “password”)
private WebElement passwordField;
@FindBy(id = “loginButton”)
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
loginButton.click();
}
}
3. Utilize Data-Driven Tests
This form of testing lets you run similar testing with several series of data, reducing redundancy and increasing test coverage. Utilize external data sources like databases, CSV, or Excel to feed test data.
Example with TestNG and Excel:
@DataProvider(name = “loginData”)
public Object[][] loginData() {
return new Object[][] {
{“user1”, “pass1”},
{“user2”, “pass2”}
};
}
@Test(dataProvider = “loginData”)
public void testLogin(String username, String password) {
LoginPage loginPage = new LoginPage(driver);
loginPage.login(username, password);
// Assertions and verifications
}
4. Use Browser Profiles
Browser profiles enable you to configure the web browser environment as per the testing needs. For instance, you can set exact preferences, add extensions, or disable notifications.
- Sample with ChromeOptions
ChromeOptions options = new ChromeOptions();
options.addArguments(“disable-notifications”);
WebDriver driver = new ChromeDriver(options);
5. Handle Alerts, Frames, and Windows
Automate connections with frames, alerts, and several windows by changing the WebDriver context properly.
- Handling Alerts:
Alert alert = driver.switchTo().alert();
alert.accept(); // or alert.dismiss();
- Switching Frames:
driver.switchTo().frame(“frameName”);
driver.switchTo().defaultContent();
- Handling Multiple Windows:
String mainWindow = driver.getWindowHandle();
for (String windowHandle : driver.getWindowHandles()) {
driver.switchTo().window(windowHandle);
// Perform actions on the new window
}
driver.switchTo().window(mainWindow);
6. Leverage Headless Browser Tests
Headless browsers enable you to conduct testing without a GUI, making the testing faster and fit for Continuous Integration/Continuous Delivery pipelines.
- Instance with Headless Chrome:
ChromeOptions options = new ChromeOptions();
options.addArguments(“–headless”);
WebDriver driver = new ChromeDriver(options);
7. Make use of Assertions Effectively
Include assertions to check the test results. This guarantees that the actual outputs match the projected results.
- Instance with JUnit Assertions
Assert.assertEquals(expectedTitle, driver.getTitle());
8. Capture Screenshots of Test Failures
Capturing screenshots for failures assists in detecting and addressing issues rapidly.
- Example:
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File(“path/to/screenshot.png”));
9. Execute Logging
Logging gives insights into the test implementation procedure and assists in diagnosing problems. Utilize logging frameworks such as SLF4J or Log4j.
- Instance with Log4j:
private static final Logger logger = LogManager.getLogger(TestClass.class);
@Test
public void testMethod() {
logger.info(“Test started”);
// Test steps
logger.info(“Test completed”);
}
10. Incorporate with CI (Continuous Integration) Tools
Incorporate your Selenium testing with CI (Continuous Integration) tools such as Travis CI, Jenkins, or CircleCI to automate the implementation of testing on each code change, guaranteeing early uncovering of issues.
Why should you consider integrating Selenium WebDriver with cloud-based platforms?
Incorporating Selenium WebDriver with cloud-centric platforms such as LambdaTest offers a more reliable and comprehensive test process. This incorporation increases the proficiencies of Selenium WebDriver, confirming comprehensive tests as well as readiness for production.
LambdaTest is a cloud-based testing platform that allows you to run Selenium WebDriver scripts on a scalable grid of real browsers and operating systems. It provides cross-browser testing in real-time, enabling you to test your web applications across various environments without needing to set up physical devices or complex configurations. This LambdaTest platform is an AI-powered test execution platform that lets you run manual and automation tests at scale across 3000+ browsers and OS combinations.
With LambdaTest, you can automate your tests, speed up your development cycles, and ensure seamless performance on a wide range of browsers and devices—all while reducing the need for local infrastructure.
Final Verdict
In short, Selenium WebDriver is the most influential automation testing tools that offers robust capabilities for cross-browser testing, accurate content management, file operations, and more. By discovering innovative use cases and following best practices, you can considerably improve the capacity and reliability of your automated testing efforts. For a robust and comprehensive test process, the inclusion of cloud-centric platforms such as LambdaTest is highly recommended. LambdaTest supports JUnit tests and Selenium tests across a plethora of actual browsers and OSs, confirming that your apps deliver a smooth user experience on all platforms and devices.